Frontend Performance Tips in Angular, React, and Vue: Optimizing Your Modern Web Applications
November 26, 2024
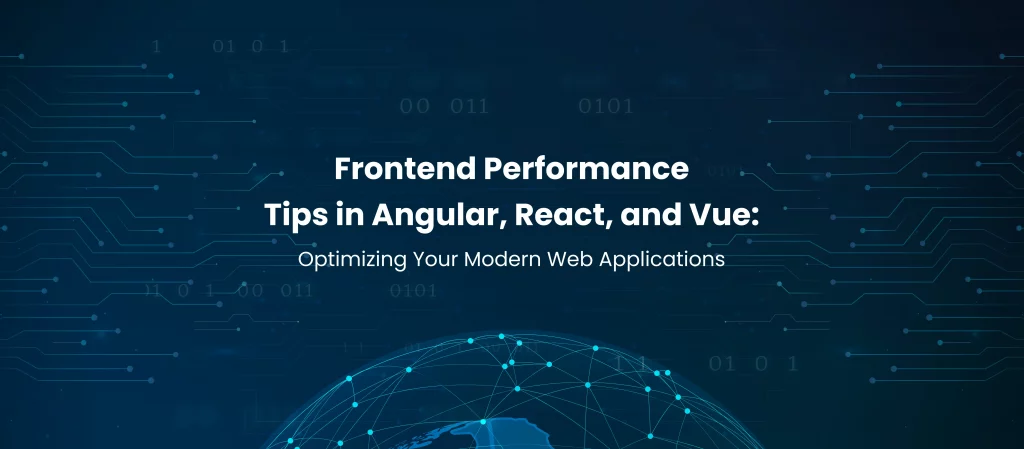
Web applications play a critical role in providing remote services and a seamless user experience. Slow load times, sluggish interactions, and an unresponsive interface can trouble users and harm your business reputation. So, optimization is needed. When building responsive web applications with frameworks like Angular, React, or Vue, make sure your are optimized for a fast, responsive user experience. Well, you can build a robust application development with these frameworks, but you need careful attention to performance-optimizing techniques. We are going to explore the essential frontend performance tips dedicated for Angular, React, and Vue to help you build faster and more efficient web applications.
Let’s dive into some of the best practices to optimize your modern web applications.
1. Code Splitting
Code splitting allows you to load only the necessary code at any given time to improve performance and user experience. Angular, React, and Vue all support code splitting, which is a technique that breaks down your application’s code into smaller, more manageable chunks. This way, only the necessary code is loaded when a user accesses a specific route or component. It will enable dynamic loading and optimize for load time.
Angular: Angular CLI has built-in support for lazy loading modules, making it easy to split the application into smaller pieces. By using the Angular Router’s `loadChildren` property, you can load modules on demand.
typescript
const routes: Routes = [
{
path: ‘feature’,
loadChildren: () => import(‘./feature/feature.module’).then(m => m.FeatureModule)
}
];
React: React uses dynamic `import()` to split code. The `React.lazy()` function allows for easy implementation of lazy loading components:
javascript:
const FeatureComponent = React.lazy(() => import(‘./FeatureComponent’));
Vue: Vue Router supports lazy loading components as well. You can import components dynamically using the `import()` syntax in your routes configuration:
javascript:
const routes = [
{
path: ‘/feature’,
component: () => import(‘./components/FeatureComponent.vue’)
}
];
Benefits: Code splitting improves performance by loading only the necessary code for the current view, reducing initial load times and bandwidth usage.
2. Tree Shaking
Tree shaking is an essential optimization technique that removes unused code from your application bundles, making them smaller, faster, and more efficient. Angular, React, and Vue all support tree shaking when using modern JavaScript bundlers like Webpack.
Angular: The Angular CLI automatically applies tree shaking when you build the application in production mode (`ng build –prod`).
React and Vue: When using Webpack for React or Vue, tree shaking is enabled in production builds. Make sure your code follows ES6 module standards for the best results.
Tip: Avoid bundling libraries that include unnecessary features. For example, when using utility libraries like Lodash, only import the functions you need:
javascript:
import { debounce } from ‘lodash/debounce’;
Benefits: Tree shaking significantly reduces the final bundle size, leading to faster load times and improved application performance.
3. Dynamic Imports
Dynamic imports, or lazy loading components on demand, are a powerful technique to improve application speed by loading components only when they are needed. As the name suggests, you can import them on the fly.
Angular: Dynamic imports are built into Angular’s lazy-loading system for modules. Use this approach for routes and features that are not critical for the initial page load.
React: React supports dynamic imports via `React.lazy()` and `Suspense` for component-level code splitting.
javascript:
const SomeComponent = React.lazy(() => import(‘./SomeComponent’));
Vue: Similar to Angular and React, Vue uses dynamic imports in its router configuration, which allows for loading components only when needed.
Benefits: Dynamic imports reduce initial load times by deferring the loading of non-essential parts of your application.
4. Pre-Fetching and Pre-Loading Resources
Once code splitting and dynamic imports are in place, pre-fetching resources that will likely be needed soon can further enhance performance. Pre-fetching allows the browser to download these resources during idle time, so they’re ready when the user needs them. With these techniques, developers can improve web performance and user satisfaction.
React and Vue: Webpack’s `PrefetchPlugin` and `PreloadPlugin` work well for both frameworks, and libraries like `react-loadable` in React or `vue-lazyload` in Vue can automate this process.
Angular: Use the `PreloadAllModules` strategy in Angular’s Router for preloading lazy-loaded modules:
RouterModule.forRoot(routes, { preloadingStrategy: PreloadAllModules })
Benefits: Pre-fetching reduces latency for user interactions by having resources readily available before they are needed.
5. Optimize Image Loading
Images are often the heaviest assets on a webpage. And they impact page load time. Optimizing their loading strategy is crucial.
Angular: Use Angular’s native support for lazy loading images by setting the `loading=”lazy”` attribute in `<img>` tags.
React and Vue: Both frameworks support third-party libraries like `react-lazyload` or `vue-lazyload` to load images only when they appear in the viewport.
General Tip: Always use modern image formats like WebP and optimize image sizes to minimize bandwidth usage.
Benefits: Lazy loading images reduces the initial loading time and bandwidth usage, ensuring that only visible images are loaded first.
6. Minification and Compression
All three frameworks support minification and compression techniques to reduce the size of JavaScript, CSS, and HTML files. These techniques will help to improve a site’s speed and SEO.
Angular: The Angular CLI automatically minifies and compresses files when building for production.
React and Vue: Webpack’s built-in plugins handle minification during production builds. Tools like Babel Minify or Terser further optimize code size.
Benefits: Minification and compression ensure that your application’s files are as small as possible, reducing the time required for the browser to download and parse them.
7. Priority-Based and Optimized Loading Sequence
Ensuring that your application loads resources in the correct order can dramatically improve perceived performance. The user will experience faster load times, higher satisfaction, and better engagement.
Angular: Angular’s `APP_INITIALIZER` can be used to load essential resources before the application is fully bootstrapped.
React and Vue: For React, you can manage resource loading using libraries like `Loadable Components`. For Vue, you can configure the router and use Vue Meta to prioritize essential resources.
Tip: Use critical CSS to load only essential styles for above-the-fold content initially, deferring the rest.
Benefits: Optimizing the loading sequence ensures that the most critical parts of the application load first, reducing time-to-interactive (TTI) and improving user experience.
8. Caching and Service Workers
Caching is a powerful way to improve performance for subsequent visits. And service workers check if the resource is cached or not, then decide whether to return the resource itself based on its programmed caching strategies. Frameworks like Angular, React, and Vue offer tools to easily integrate caching and service workers.
Angular: The Angular Service Worker module provides a straightforward way to add PWA capabilities, including caching strategies.
React: Libraries like `Workbox` make it easy to set up service workers in React projects.
Vue: The `@vue/pwa` plugin provides service worker integration for Vue applications, offering caching and offline support.
Benefits: Caching can drastically reduce load times for repeat users, while service workers enable offline functionality, creating a more resilient user experience.
9. Tree Shaking and Dead Code Elimination
Dead code elimination, often referred to as tree shaking, is essential for reducing the final build size. They help in reducing the size by identifying and removing unused code, resulting in faster loading times and improved performance.
Angular: Angular CLI’s production build automatically removes dead code.
React and Vue: With Webpack and Babel, you can set up tree shaking to eliminate unnecessary code in both React and Vue applications.
Benefits: By removing unused code, you can reduce the overall bundle size, leading to faster loading times.
These are the 9 best practices for optimizing your modern web application for faster load times and improved performance. By incorporating these practices, you are eliminating unnecessary code, loading the necessary components, and improving the overall performance. This will lead to a superior user experience, better interaction, and faster load time.
Keep reading about
LEAVE A COMMENT
We really appreciate your interest in our ideas. Feel free to share anything that comes to your mind.