Exploring Angular Standalone Components: A New Era of Simplified Modular Design
November 19, 2024
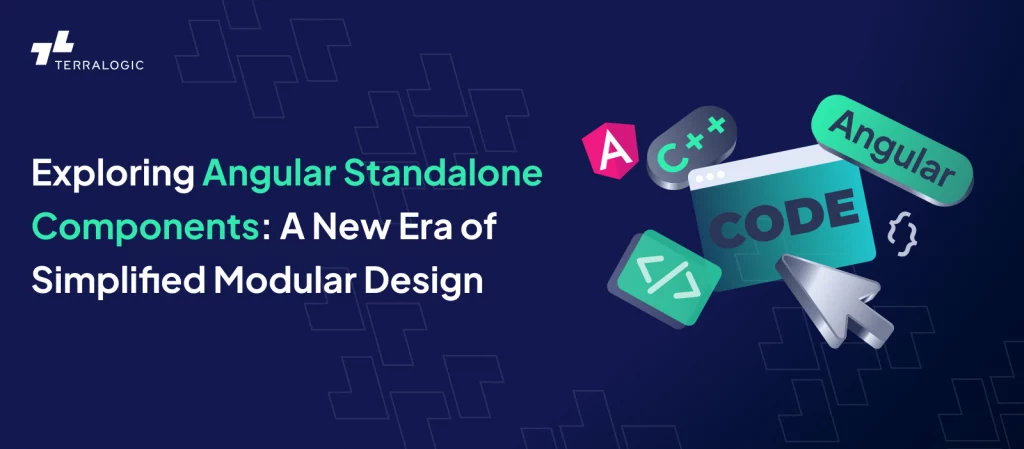
Angular has been on a developmental trajectory in the last couple of years, introducing newer enhancements to the framework. Newer releases have particularly been affected by a change that has become one of the most viable and significant changes in Angular—the provision of Angular standalone components, which pushes Angular towards more modularity and simplicity for the developers. This shift aligns with the concept of module-free Angular components and embraces an Angular module-less architecture, leading to the rethinking of how components should be designed. It makes it possible to build, test, and scale applications effectively without reliance on NgModules, which has been the norm for most developers.
What are standalone components?
Standalone components are a step toward “modular independence” in Angular. Previously, in the architecture of the Angular application, components, services, and directives were contained in a separate NgModule, the unit of organization that allowed Angular to manage dependencies. The Angular ecosystem benefited greatly from this strategy, which allowed us to construct the projects consistently and cohesively while adding more verbosity and complexity—particularly in large-scale or micro-frontend applications. Standalone components provide functionality to operate independently without dependencies on NgModules at a component level, therefore enabling each component to be self-sufficient and responsible for its own dependencies.
Why Standalone Components Matter
The application architecture is eased by Angular standalone components that allow developers to instantiate and use components without the need to declare an enclosing NgModule. This approach, integral to modern Angular component design, reduces boilerplate code, simplifying the development process, particularly for projects requiring customization, such as micro-frontends, modular applications, or libraries. In a standalone component, you only specify the dependencies intended for that particular component, keeping the dependency tree cleaner and clearer, which also improves tree-shakability. For teams looking to optimize their projects, it is beneficial to Angular migrate to standalone components for better modularity and streamlined development workflows.
Benefits of Angular Standalone Components
- Reduced Complexity: Thanks to standalone components, “module scaffolding” is no longer necessary because this type of component transforms the number of files or declarations required to handle a specific component while incorporating a single file and a single declaration.
- Enhanced Performance: Standalone components are loaded independently as needed. This allows Angular to optimize and tree-shake further, meaning faster load times and a more responsive user experience.
- Improved Code Organization: Standalone components are the best way to separate out your logic, meaning every single component is independent and can be reused without any module configuration. It helps optimize code and provides better performance.
- Facilitates Micro-frontend architectures: Modular and independent development and deployment. Spinibell standalone components are good for micro-frontend applications that require isolated Javascript modules as independent elements inside the final build step instead of being part of a large shared bundle.
Practical Applications and Usage
These standalone components can easily work in both module-based and standalone setups, which means that you are free to mix and match them into an existing application. So we should use them in the form of reusable, isolated components such as widgets, popups, or other utility components. This makes a standalone component the ideal choice for situations where you need something lightweight, either independent or partially isolated.
Dependency Management in Angular Standalone Components
Standalone components dramatically change and enable specific reliance management. Instead of relying on a root NgModule to provide dependencies throughout your app, each standalone component specifies its imports and dependencies explicitly. This provides the component with more control and at the same time simplifies testing as developers can now load only required dependencies instead of having one big loaded test module, which is now causing modularisation between tests so that it takes less setup timing.
Real-World Benefits: Lazy Loading and Reusability
One of the most useful features that standalone components bring to Angular is making lazy loading a no-brainer. When a standalone capable component is lazy loaded, it requires only minimal set-up as there isn’t any separate module wrapping the logic of the component. This comes in especially handy for applications that are focused on performance optimization and need to cut down on loading times. Lazy loading of what we need to load will help Angular applications show the page as quickly as possible and make its experience smoother.
Also, because standalone components are isolated (contain everything you need within themselves), they are the most reusable components. Standalone components can be reused in different parts of an application or even across several applications without depending on any module configuration. This makes standalone components a perfect fit for component libraries where portability, reusability, and isolation are critical.
Considerations and Best Practices
Despite all the benefits of using standalone components, they come with a cost, and that is changing your mindset to adopt them in practicality. Remember, although standalone components allow more flexibility, it comes down to being careful about dependency management. Having a lot of things means that your component inherits them in the first place and they are not really up for it. If you add some extra non-related dependencies to this container, it’s more complex than before, making features harder.
Backward compatibility is another important factor to consider. Stride components are also compatible with traditional NgModules and can be introduced incrementally. This would be useful for teams that operate on a large codebase and cannot afford to migrate the whole thing at once. Isolated or utility components are often the best way to ease the transition to standalone components without doing a full-scale refactor.
Looking Ahead: Angular’s Future with Standalone Components
It´s a big step forward for Angular, which shows how this framework is becoming more flexible & modern today. Thanks to its modular and lightweight approach, Angular allows developers to build applications that are faster, easier to maintain, and scale well for a large user base. There will be more frameworks and tools built on top of the Angular modular setup, opening the door to new ideas people haven’t thought of before in a frontend technology.
Conclusion
Angular standalone components represent a significant shift in how we build and organize apps. They provide an effective, adaptable platform for developing simplified applications that value modularity and independence. For developers aiming to simplify their Angular apps and enhance reusability, standalone components Angular offers a compelling option that is both innovative and practical. By leveraging Angular standalone components, developers can streamline their projects, making it easier to manage and scale. If you’re considering a transition, the decision to Angular migrate to standalone components is a forward-thinking approach to modern application development.
FAQs for Angular Standalone Components
1. What is Angular Standalone Components
Angular Standalone Components are a new feature introduced in Angular to simplify application development by making components self-contained and modular. Unlike traditional components that rely on NgModules for declarations, standalone components can directly import necessary Angular features, reducing complexity and improving reusability. They enable developers to build more efficient, adaptable, and scalable applications with minimal dependencies.
2. What are Angular standalone components?
Angular standalone components are a feature in Angular that allows developers to create self-contained, reusable components without relying on NgModules for configuration. They simplify the app structure, improve modularity, and enhance code maintainability, making building and scaling modern applications easier.
Here’s a basic example of how to create and use Angular standalone components:
i. Creating a Standalone Component
In Angular, standalone components are defined with the standalone: true
property in the component metadata. This makes the component self-contained and independent of an NgModule
.
import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-standalone’,
template: `<h1>Hello from Standalone Component!</h1>`,
standalone: true,
styleUrls: [‘./standalone.component.css’]
})
export class StandaloneComponent {
// You can add logic here if needed
}
ii. Using a Standalone Component in Another Component
To use the standalone component, you can directly import it in the component where you need it, without needing to include it in any module.
import { Component } from ‘@angular/core’;
import { StandaloneComponent } from ‘./standalone.component’;
@Component({
selector: ‘app-main’,
template: `
<div>
<h2>Main Component</h2>
<app-standalone></app-standalone> <!– Using the standalone component here –>
</div>
`,
standalone: true,
imports: [StandaloneComponent] // Importing the standalone component directly
})
export class MainComponent {
// Logic for the main component
}
iii. Using Standalone Component with Router
Standalone components can also be used with Angular’s router. Here’s how you define a route for a standalone component:
import { Route } from ‘@angular/router’;
import { StandaloneComponent } from ‘./standalone.component’;
const routes: Route[] = [
{
path: ‘standalone’,
component: StandaloneComponent
}
];
iv. Standalone Component with Services
You can also inject services into a standalone component just like a regular component. Here’s an example of injecting a service:
import { Component } from ‘@angular/core’;
import { DataService } from ‘./data.service’;
@Component({
selector: ‘app-standalone’,
template: `<h1>{{data}}</h1>`,
standalone: true,
providers: [DataService]
})
export class StandaloneComponent {
data: string;
constructor(private dataService: DataService) {
this.data = dataService.getData();
}
}
In this setup, DataService is a simple Angular service used to fetch data. The StandaloneComponent is self-contained, and no need to add it to an NgModule.
3. How to use standalone component in another non-standalone component in Angular?
To use a standalone component in a non-standalone component, import the standalone component into the NgModule
of the non-standalone component. Add the standalone component to the declarations
array of the module where the non-standalone component resides.
4. What is @NgModule in Angular?
@NgModule
is a decorator in Angular that defines a module, which is a container for components, directives, pipes, and services. It organizes the application into cohesive blocks, managing imports, declarations, and providers to configure the injector and component rendering.
5. What happens if I forget to import the standalone component?
Angular will throw an error like:
‘app-standalone’ is not a known element
Keep reading about
LEAVE A COMMENT
We really appreciate your interest in our ideas. Feel free to share anything that comes to your mind.